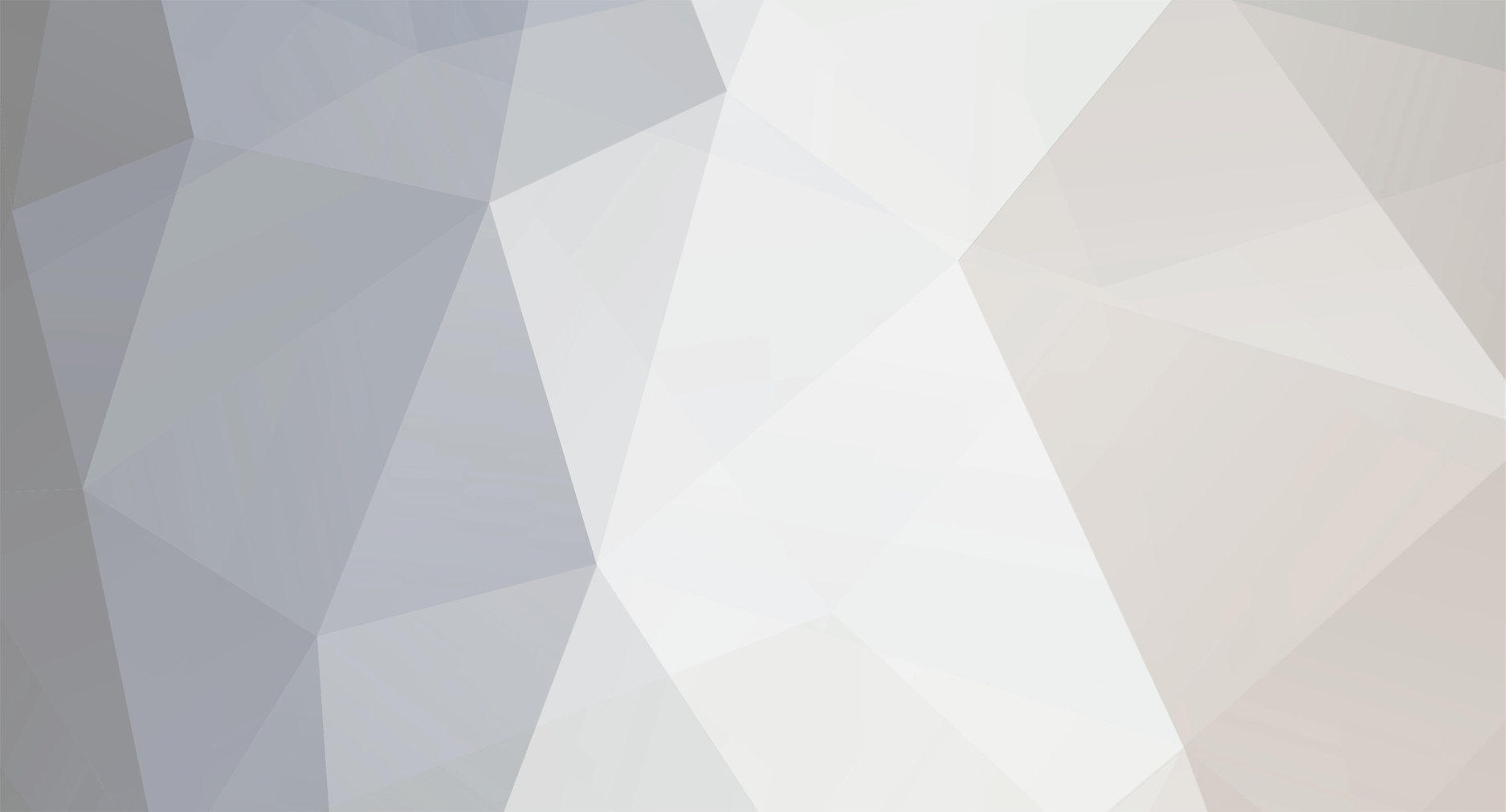
crteensy
Members-
Posts
111 -
Joined
-
Last visited
Content Type
Forums
Store
Downloads
Blogs
Everything posted by crteensy
-
It's not quite clear to me how I would displace a widget within its parent container. I have the following code, but the widget always ends up in one of the corners after this has been done a few times with dx = dy = 0: int dx = 0;//round(c4*it->force_x); int dy = 0;//round(c4*it->force_y); coord_t x = gwinGetScreenX(lblHandle) + dx; coord_t y = gwinGetScreenY(lblHandle) + dy; x -= gwinGetScreenX(ghContainer); y -= gwinGetScreenY(ghContainer); uint8_t border = 2; x -= border; y -= border; printf("d = %03d %03d, c = %03d %03d\n", dx, dy, x, y); gwinMove(lblHandle, x, y); with border < 2 it's in the lower right corner, with border = 2 it's in the upper left. I can't find a coordinate combo that does what I want. You already said that gwinMove() is not thoroughly tested - might that be the culprit? Working with the container's inner height and width might also work, but I expect to get off-by-one errors with that, too.
-
My current workaround is this: // A mouse move has occurred static void MouseMove(GWidgetObject *gw, coord_t x, coord_t y) { if (((GDragLabelObject*)gw)->beingDragged == TRUE) { GDragLabelObject* p = (GDragLabelObject*)gw; p->moved = TRUE; // gwinMove((GWindowObject *)gw, p->w.g.x+x-p->hotspot_x, p->w.g.y+y-p->hotspot_y); coord_t winx = gwinGetScreenX((GWindowObject*)gw); coord_t winy = gwinGetScreenY((GWindowObject*)gw); printf("winx = %d, winy = %d\n", winx, winy); #if(GWIN_NEED_CONTAINERS) if (gw->g.parent) { GWindowObject* parent = gw->g.parent; winx -= parent->x; winy -= parent->y; printf(" relx = %d, rely = %d\n", winx, winy); } #endif gwinMove((GWindowObject *)gw, winx+x-p->hotspot_x, winy+y-p->hotspot_y); } else { printf("empty move\n"); } } is there something like gwinGetRelX() and gwinGetRelY() functions or maybe even a more elegant way that I haven't found?
-
Also, when the label is dragged across one of the buttons, the button is "damaged". It should be redrawn at least after the label is placed at its destination (I solved that by now, when the label is placed after being moved it calls gwinRedrawDisplay()).
-
Incredibly sketchy and hacked together from button and label code, but it works, sort of. The code below does more than just show a draggable label because I added the label code to an existing draft. It's kind of a main menu with two buttons, one of which opens a sub menu with a "back" button. These work fine. The magic is inside the MouseDown, MouseUp and MouseMove functions. Once clicked a hotspot is stored and while the mouse is down the label is moved relative to this hotspot. There is one problem, though: If the label's parent container doesn't cover the whole screen (see cwidth and cheight in createWidgets()), the calculated coordinates for gwinMove are wrong. How can I move the label relative to its parent? #include #include "gfx.h" #include "src/gwin/gwin_class.h" // macros to assist in data type conversions #define gh2obj ((GLabelObject *)gh) #define gw2obj ((GLabelObject *)gw) // flags for the GLabelObject #define GLABEL_FLG_WAUTO (GWIN_FIRST_CONTROL_FLAG << 0) #define GLABEL_FLG_HAUTO (GWIN_FIRST_CONTROL_FLAG << 1) #define GLABEL_FLG_BORDER (GWIN_FIRST_CONTROL_FLAG << 2) static GHandle ghMainContainer; static GHandle ghSubContainer; static GHandle ghButton1; static GHandle ghButton1Back; static GHandle ghButton2; static GHandle ghDragLabel; static GWidgetStyle myStyle = { Black, // background {White, Orange, Orange, Black}, // enabled (text edge fill progress) {White, Gray, Gray, Black}, // disabled {White, Gray, Orange, Black} // pressed }; typedef struct GDragLabelObject { GWidgetObject w; coord_t hotspot_x; coord_t hotspot_y; bool_t beingDragged; } GDragLabelObject; #if GINPUT_NEED_MOUSE // A mouse down has occurred over the button static void MouseDown(GWidgetObject *gw, coord_t x, coord_t y) { (void) x; (void) y; GDragLabelObject* p = (GDragLabelObject*)gw; p->beingDragged = TRUE; p->hotspot_x = x; p->hotspot_y = y; } // A mouse up has occurred (it may or may not be over the button) static void MouseUp(GWidgetObject *gw, coord_t x, coord_t y) { (void) x; (void) y; GDragLabelObject* p = (GDragLabelObject*)gw; p->beingDragged = FALSE; } // A mouse move has occurred static void MouseMove(GWidgetObject *gw, coord_t x, coord_t y) { if (((GDragLabelObject*)gw)->beingDragged == TRUE) { GDragLabelObject* p = (GDragLabelObject*)gw; gwinMove((GWindowObject *)gw, p->w.g.x+x-p->hotspot_x, p->w.g.y+y-p->hotspot_y); } } #endif static void gwinDragLabelDefaultDraw(GWidgetObject *gw, void *param); static const gwidgetVMT dragLabelVMT = { { "DragLabel", // The classname sizeof(GDragLabelObject), // The object size _gwidgetDestroy, // The destroy routine _gwidgetRedraw, // The redraw routine 0, // The after-clear routine }, gwinDragLabelDefaultDraw, // The default drawing routine #if GINPUT_NEED_MOUSE { MouseDown, // Process mouse down events MouseUp, // Process mouse up events MouseMove, // Process mouse move events (NOT USED) }, #endif #if GINPUT_NEED_TOGGLE { 0, 0, 0, 0, 0, // No toggles }, #endif #if GINPUT_NEED_DIAL { 0, 0, 0, 0, // No dials }, #endif }; // simple: single line with no wrapping static coord_t getwidth(const char *text, font_t font, coord_t maxwidth) { (void) maxwidth; return gdispGetStringWidth(text, font)+2; // Allow one pixel of padding on each side } // simple: single line with no wrapping static coord_t getheight(const char *text, font_t font, coord_t maxwidth) { (void) text; (void) maxwidth; return gdispGetFontMetric(font, fontHeight); } static void gwinDragLabelDefaultDraw(GWidgetObject *gw, void *param) { coord_t w, h; color_t c; (void) param; // is it a valid handle? if (gw->g.vmt != (gwinVMT *)&dragLabelVMT) return; w = (gw->g.flags & GLABEL_FLG_WAUTO) ? getwidth(gw->text, gw->g.font, gdispGGetWidth(gw->g.display) - gw->g.x) : gw->g.width; h = (gw->g.flags & GLABEL_FLG_HAUTO) ? getheight(gw->text, gw->g.font, gdispGGetWidth(gw->g.display) - gw->g.x) : gw->g.height; c = (gw->g.flags & GWIN_FLG_SYSENABLED) ? gw->pstyle->enabled.text : gw->pstyle->disabled.text; if (gw->g.width != w || gw->g.height != h) { gwinResize(&gw->g, w, h); return; } #if GWIN_LABEL_ATTRIBUTE if (gw2obj->attr) { gdispGFillStringBox(gw->g.display, gw->g.x, gw->g.y, gw2obj->tab, h, gw2obj->attr, gw->g.font, c, gw->pstyle->background, justifyLeft); gdispGFillStringBox(gw->g.display, gw->g.x + gw2obj->tab, gw->g.y, w-gw2obj->tab, h, gw->text, gw->g.font, c, gw->pstyle->background, justifyLeft); } else gdispGFillStringBox(gw->g.display, gw->g.x, gw->g.y, w, h, gw->text, gw->g.font, c, gw->pstyle->background, justifyLeft); #else gdispGFillStringBox(gw->g.display, gw->g.x, gw->g.y, w, h, gw->text, gw->g.font, c, gw->pstyle->background, justifyLeft); #endif // render the border (if any) if (gw->g.flags & GLABEL_FLG_BORDER) gdispGDrawBox(gw->g.display, gw->g.x, gw->g.y, w, h, (gw->g.flags & GWIN_FLG_SYSENABLED) ? gw->pstyle->enabled.edge : gw->pstyle->disabled.edge); } GHandle gwinGDragLabelCreate(GDisplay *g, GDragLabelObject *gw, GWidgetInit *pInit) { uint16_t flags = 0; // auto assign width if (pInit->g.width <= 0) { flags |= GLABEL_FLG_WAUTO; pInit->g.width = getwidth(pInit->text, gwinGetDefaultFont(), gdispGGetWidth(g) - pInit->g.x); } // auto assign height if (pInit->g.height <= 0) { flags |= GLABEL_FLG_HAUTO; pInit->g.height = getheight(pInit->text, gwinGetDefaultFont(), gdispGGetWidth(g) - pInit->g.x); } if (!(gw = (GDragLabelObject *)_gwidgetCreate(g, &gw->w, pInit, &dragLabelVMT))) return 0; gwinSetVisible((GHandle)gw, pInit->g.show); return (GHandle)gw; } #define gwinDragLabelCreate(gb, pInit) gwinGDragLabelCreate(GDISP, gb, pInit) static void createWidgets(void) { uint8_t btnSize = 48; uint8_t btnDist = btnSize/2; coord_t height = gdispGetHeight(); coord_t width = gdispGetWidth(); coord_t cheight = height/2; coord_t cwidth = width/2; GWidgetInit wi; // Apply some default values for GWIN gwinWidgetClearInit(&wi); // Apply the container parameters wi.g.show = FALSE; wi.g.width = cwidth; wi.g.height = cheight; wi.g.x = (width - cwidth)/2; wi.g.y = (height - cheight)/2; wi.text = "Main Container"; ghMainContainer = gwinContainerCreate(0, &wi, 0);//GWIN_CONTAINER_BORDER); wi.g.show = TRUE; wi.text = "Bake Container"; wi.g.show = FALSE; ghSubContainer = gwinContainerCreate(0, &wi, 0);//GWIN_CONTAINER_BORDER); // Apply the button parameters wi.g.width = btnSize; wi.g.height = btnSize; wi.g.x = cwidth/2 - btnSize - btnDist/2; wi.g.y = cheight/2-btnSize/2; wi.text = "Button 1"; wi.g.parent = ghMainContainer; wi.g.show = TRUE; ghButton1 = gwinButtonCreate(0, &wi); // Apply the button parameters wi.g.x = cwidth/2 + btnDist/2; wi.text = "Button 2"; wi.g.parent = ghMainContainer; ghButton2 = gwinButtonCreate(0, &wi); // Apply the button parameters wi.g.x = 3*cwidth/4; wi.g.y = 3*cheight/4; wi.text = "drag me"; wi.g.parent = ghMainContainer; ghDragLabel = gwinDragLabelCreate(0, &wi); wi.g.x = btnDist; wi.g.y = btnDist; wi.text = "Back"; wi.g.parent = ghSubContainer; ghButton1Back = gwinButtonCreate(0, &wi); // Make the container become visible - therefore all its children // become visible as well gwinHide(ghSubContainer); gwinShow(ghMainContainer); } static GListener gl; int main(void) { // Initialize the display gfxInit(); // Set the widget defaults gwinSetDefaultFont(gdispOpenFont("*")); gwinSetDefaultStyle(&myStyle, FALSE); // gwinSetDefaultStyle(&WhiteWidgetStyle, FALSE); gdispClear(Black); // Create the widget createWidgets(); geventListenerInit(&gl); gwinAttachListener(&gl); GEvent* pe; while(1) { pe = geventEventWait(&gl, TIME_INFINITE); switch(pe->type) { case GEVENT_GWIN_BUTTON: if (((GEventGWinButton*)pe)->gwin == ghButton1) { gwinHide(ghMainContainer); gwinShow(ghSubContainer); } else if (((GEventGWinButton*)pe)->gwin == ghButton1Back) { gwinHide(ghSubContainer); gwinShow(ghMainContainer); } break; default: break; } } return 0; }
-
gwinMove works, I've tested that with a label. So I'll see if I can get it to work with custom code and report back.
-
Is it possible to drag a widget around? Currently I just need a label, though I'm not yet sure if that will change in the future. I'd use this in a force directed graph demo. The buttonVMT table in gwin_button.c seems to indicate that dragging is not possible because there's no function that handles mouse move events (I have no idea if I'm looking in the right place!)
-
I can do that, but as it is now it shares a common header (register address definitions and bit masks) with the RA8875 display driver. Would you like a separate header with only the touch panel definitions? This should be done right before anyone starts using it.
-
Apparently -O1 is good as well. Also reducing the timer thread working area to 512 bytes didn't hurt. I guess I'll just leave it at that...
-
Seems to run ok with -O0. I'm a bit disappointed by that, but I'll see if I hit any speed or size issues (probably not). The label example has been running stable for about 550 seconds until I decided to try something different. The touch_driver_test works (a bit clumsy, but that's my crappy calibration and I was probably drunk while I did that) as well as touch_raw_readings. Great!
-
That's a bummer. I can't test it now, but -O0 results in significantly larger code (~58 kB vs ~40 kB) and it might even be slower (I'd have to test, though). The biggest problem is that I have to modify my linker script because the startup code is now too large, which might have other implications that I'm not aware of. If ugfx works for me with -O0 I can turn it into a library (or several) for linking and compile my other code with other optimization settings. To be honest I didn't encounter optimizer problems with -O2 and -Os until now.
-
I can rearrange my code but I really don't think that this will solve my problem. Of course, better separation between driver and board is needed for portability, but I'm not facing a portability issue here (or am I?). As my display has a built-in touch panel controller such a separation will probably end with a mess anyway... I've posted compiler details in my widget thread: viewtopic.php?f=23&t=198
-
I had the stack size set to zero only to see if it would compile with that. In the config I posted later it's set to 2048, and even without the #define the application hangs. My compiler is arm-none-eabi-gcc (the one that comes with teensyduino) with this configuration: > arm-none-eabi-gcc -v Using built-in specs. COLLECT_GCC=arm-none-eabi-gcc COLLECT_LTO_WRAPPER=/users/crteensy/builds/arduino/arduino-1.0.6/hardware/tools/arm/bin/../lib/gcc/arm-none-eabi/4.8.4/lto-wrapper Target: arm-none-eabi Configured with: /home/build/toolchain/gcc-arm-none-eabi-4_8-2014q3-20140805/src/gcc/configure --target=arm-none-eabi --prefix=/home/build/toolchain/gcc-arm-none-eabi-4_8-2014q3-20140805/install-native --libexecdir=/home/build/toolchain/gcc-arm-none-eabi-4_8-2014q3-20140805/install-native/lib --infodir=/home/build/toolchain/gcc-arm-none-eabi-4_8-2014q3-20140805/install-native/share/doc/gcc-arm-none-eabi/info --mandir=/home/build/toolchain/gcc-arm-none-eabi-4_8-2014q3-20140805/install-native/share/doc/gcc-arm-none-eabi/man --htmldir=/home/build/toolchain/gcc-arm-none-eabi-4_8-2014q3-20140805/install-native/share/doc/gcc-arm-none-eabi/html --pdfdir=/home/build/toolchain/gcc-arm-none-eabi-4_8-2014q3-20140805/install-native/share/doc/gcc-arm-none-eabi/pdf --enable-languages=c,c++ --enable-plugins --disable-decimal-float --disable-libffi --disable-libgomp --disable-libmudflap --disable-libquadmath --disable-libssp --disable-libstdcxx-pch --disable-nls --disable-shared --disable-threads --disable-tls --with-gnu-as --with-gnu-ld --with-newlib --with-headers=yes --with-python-dir=share/gcc-arm-none-eabi --with-sysroot=/home/build/toolchain/gcc-arm-none-eabi-4_8-2014q3-20140805/install-native/arm-none-eabi --build=x86_64-linux-gnu --host=x86_64-linux-gnu --with-gmp=/home/build/toolchain/gcc-arm-none-eabi-4_8-2014q3-20140805/build-native/host-libs/usr --with-mpfr=/home/build/toolchain/gcc-arm-none-eabi-4_8-2014q3-20140805/build-native/host-libs/usr --with-mpc=/home/build/toolchain/gcc-arm-none-eabi-4_8-2014q3-20140805/build-native/host-libs/usr --with-isl=/home/build/toolchain/gcc-arm-none-eabi-4_8-2014q3-20140805/build-native/host-libs/usr --with-cloog=/home/build/toolchain/gcc-arm-none-eabi-4_8-2014q3-20140805/build-native/host-libs/usr --with-libelf=/home/build/toolchain/gcc-arm-none-eabi-4_8-2014q3-20140805/build-native/host-libs/usr --with-host-libstdcxx='-static-libgcc -Wl,-Bstatic,-lstdc++,-Bdynamic -lm' --with-pkgversion='GNU Tools for ARM Embedded Processors' --with-multilib-list=armv6-m,armv7-m,armv7e-m,armv7-r Thread model: single gcc version 4.8.4 20140725 (release) [ARM/embedded-4_8-branch revision 213147] (GNU Tools for ARM Embedded Processors) Is it possible that it's a bug caused by some optimization setting? I'll try other settings later today.
-
The label example from the wiki also hangs in the main loop after a while (about two minutes). When I add a second label it doesn't even get past the first iteration: #include #include #include void setup() { // while(!Serial.available()); Serial.println("Start"); SPI.begin(); // Initialize and clear the display gfxInit(); // draw(); } static GHandle ghLabel1; static GHandle ghLabel2; static void createWidgets(void) { GWidgetInit wi; // Apply some default values for GWIN wi.customDraw = 0; wi.customParam = 0; wi.customStyle = 0; wi.g.show = TRUE; // Apply the label parameters wi.g.y = 10; wi.g.x = 10; wi.g.width = 100; wi.g.height = 20; wi.text = "Label 1"; // Create the actual label ghLabel1 = gwinLabelCreate(NULL, &wi); wi.g.x = 400; wi.g.y = 250; ghLabel2 = gwinLabelCreate(NULL, &wi); } static char buf[20]; void loop() { // Set the widget defaults gwinSetDefaultFont(gdispOpenFont("UI2")); gwinSetDefaultStyle(&WhiteWidgetStyle, FALSE); gdispClear(White); // create the widget createWidgets(); while(1) { snprintf(buf, 20, "%" PRIu32, millis()/1000); gwinSetText(ghLabel2, buf, TRUE); gwinSetText(ghLabel1, "This is some text", TRUE); gfxSleepMilliseconds(1000); gwinSetText(ghLabel1, "Aaaand some other text", TRUE); gfxSleepMilliseconds(1000); } } so I end up with "Aaaand some other text" in the upper left corner and "0" in the lower right.
-
Above problem might be related to threading, see my compiling with widgets thread
-
When I try to run the touch example my system gets stuck at the call to gfxSleepMilliseconds(100) in the main loop. I've added debugging messages before and after that line, but the output that comes after that line is not shown. That might be related to threading because the gfxSleepMilliseconds function calls gfxYield() and I haven't followed things further from there because I have no on-chip debugging. This is my gfxconf: #ifndef _GFXCONF_H #define _GFXCONF_H #define GFX_USE_OS_RAW32 TRUE #define GFX_NO_OS_INIT TRUE #define GFX_USE_GDISP TRUE #define GDISP_NEED_CONTROL TRUE #define GDISP_NEED_CIRCLE TRUE #define GDISP_NEED_TEXT TRUE //#define GDISP_INCLUDE_FONT_UI1 TRUE #define GDISP_INCLUDE_FONT_UI2 TRUE //#define GDISP_INCLUDE_FONT_DEJAVUSANS16 TRUE #define GDISP_INCLUDE_FONT_FIXED_7X14 TRUE #define GDISP_INCLUDE_FONT_FIXED_5X8 TRUE #define GDISP_INCLUDE_USER_FONTS TRUE #define GDISP_NEED_VALIDATION TRUE #define GDISP_NEED_CLIP TRUE #define GDISP_NEED_TEXT TRUE #define GDISP_NEED_MULTITHREAD TRUE #define GDISP_NEED_STARTUP_LOGO TRUE #define GFX_USE_GWIN TRUE #define GWIN_NEED_WINDOWMANAGER TRUE #define GWIN_NEED_WIDGET TRUE #define GWIN_NEED_LABEL TRUE #define GWIN_NEED_CONSOLE TRUE /////////////////////////////////////////////////////////////////////////// // GEVENT // /////////////////////////////////////////////////////////////////////////// /////////////////////////////////////////////////////////////////////////// // GTIMER // /////////////////////////////////////////////////////////////////////////// #define GFX_USE_GTIMER TRUE #define GTIMER_THREAD_WORKAREA_SIZE 2048 /////////////////////////////////////////////////////////////////////////// // GQUEUE // /////////////////////////////////////////////////////////////////////////// /////////////////////////////////////////////////////////////////////////// // GINPUT // /////////////////////////////////////////////////////////////////////////// #define GFX_USE_GINPUT TRUE #define GINPUT_NEED_MOUSE TRUE #define GINPUT_TOUCH_STARTRAW TRUE
-
Good, so I have these board files: gmouse_lld_RA8875_board.h: #ifndef _LLD_GMOUSE_RA8875_BOARD_H #define _LLD_GMOUSE_RA8875_BOARD_H #include "RA8875_regs.h" // Resolution and Accuracy Settings #define GMOUSE_RA8875_PEN_CALIBRATE_ERROR 8 #define GMOUSE_RA8875_PEN_CLICK_ERROR 6 #define GMOUSE_RA8875_PEN_MOVE_ERROR 4 #define GMOUSE_RA8875_FINGER_CALIBRATE_ERROR 14 #define GMOUSE_RA8875_FINGER_CLICK_ERROR 18 #define GMOUSE_RA8875_FINGER_MOVE_ERROR 14 #define GMOUSE_RA8875_Z_MIN 0 // The minimum Z reading #define GMOUSE_RA8875_Z_MAX 1 // The maximum Z reading #define GMOUSE_RA8875_Z_TOUCHON 1 // Values between this and Z_MAX are definitely pressed #define GMOUSE_RA8875_Z_TOUCHOFF 0 // Values between this and Z_MIN are definitely not pressed // How much extra data to allocate at the end of the GMouse structure for the board's use #define GMOUSE_RA8875_BOARD_DATA_SIZE 0 #ifdef __cplusplus extern "C" { #endif // __cplusplus extern void ra8875_acquire_bus(); extern void ra8875_release_bus(); extern void ra8875_write_index(uint8_t index); extern void ra8875_write_data(uint8_t data); extern uint8_t ra8875_read_data(); static bool_t init_board(GMouse *m, unsigned driverinstance) { return TRUE; } static bool_t read_xyz(GMouse *m, GMouseReading *prd) { ra8875_acquire_bus(); ra8875_write_index(RA8875_INTC2); uint8_t INTC2 = ra8875_read_data(); uint8_t pending = INTC2 & RA8875_INTCx_TP; prd->buttons = 0; prd->z = GMOUSE_RA8875_Z_MIN; if (pending) { ra8875_write_index(RA8875_TPXH); uint8_t touchx = ra8875_read_data(); ra8875_write_index(RA8875_TPYH); uint8_t touchy = ra8875_read_data(); // clear touch flag ra8875_write_index(RA8875_INTC2); ra8875_write_data(RA8875_INTCx_TP); static const int16_t xr = 20; // 16 static const int16_t xl = 240; // 240 static const int16_t yt = 220; // 220 static const int16_t yb = 35; // 35 coord_t x = (480*(touchx-xl))/(xr-xl); coord_t y = (272*(touchy-yt))/(yb-yt); prd->x = x; prd->y = y; prd->z = GMOUSE_RA8875_Z_MAX; prd->buttons = GINPUT_TOUCH_PRESSED; } ra8875_release_bus(); return TRUE; } #endif /* _LLD_GMOUSE_RA8875_BOARD_H */ gmouse_lld_RA8875.c: #include "gfx.h" #if GFX_USE_GINPUT && GINPUT_NEED_MOUSE #define GMOUSE_DRIVER_VMT GMOUSEVMT_RA8875 #include "src/ginput/ginput_driver_mouse.h" // Get the hardware interface #include "gmouse_lld_RA8875_board.h" const GMouseVMT const GMOUSE_DRIVER_VMT[1] = {{ { GDRIVER_TYPE_TOUCH, GMOUSE_VFLG_TOUCH//|GMOUSE_VFLG_CALIBRATE|GMOUSE_VFLG_CAL_TEST |GMOUSE_VFLG_ONLY_DOWN|GMOUSE_VFLG_POORUPDOWN, // Extra flags for testing only //GMOUSE_VFLG_DEFAULTFINGER|GMOUSE_VFLG_CAL_EXTREMES - Possible //GMOUSE_VFLG_NOPOLL|GMOUSE_VFLG_DYNAMICONLY|GMOUSE_VFLG_SELFROTATION|GMOUSE_VFLG_CAL_LOADFREE - unlikely sizeof(GMouse) + GMOUSE_RA8875_BOARD_DATA_SIZE, _gmouseInitDriver, _gmousePostInitDriver, _gmouseDeInitDriver }, GMOUSE_RA8875_Z_MAX, // z_max GMOUSE_RA8875_Z_MIN, // z_min GMOUSE_RA8875_Z_TOUCHON, // z_touchon GMOUSE_RA8875_Z_TOUCHOFF, // z_touchoff { // pen_jitter GMOUSE_RA8875_PEN_CALIBRATE_ERROR, // calibrate GMOUSE_RA8875_PEN_CLICK_ERROR, // click GMOUSE_RA8875_PEN_MOVE_ERROR // move }, { // finger_jitter GMOUSE_RA8875_FINGER_CALIBRATE_ERROR, // calibrate GMOUSE_RA8875_FINGER_CLICK_ERROR, // click GMOUSE_RA8875_FINGER_MOVE_ERROR // move }, init_board, // init 0, // deinit read_xyz, // get 0, // calsave 0 // calload }}; #endif /* GFX_USE_GINPUT && GINPUT_NEED_MOUSE */ The raw touch reading example gets to the point where it displays the second line, like 4294940576, 8191, z=0 B=0x0000 but that line never changes. I have tested the touch reading code before and I'm confident that it works. The board's read_xyz() doesn't seem to get called as I should see activity on the SPI.
-
The label example compiles and seems to run fine. I don't know if that is a good sign or not, given that my thread working area is still set to zero.
-
So that's a black box for me and I'll only complain if it blows up my stack. How far can I go down with GTIMER_THREAD_WORKAREA_SIZE (that's the one, isn't it)? I now have /////////////////////////////////////////////////////////////////////////// // GTIMER // /////////////////////////////////////////////////////////////////////////// //#define GFX_USE_GTIMER FALSE #define GTIMER_THREAD_WORKAREA_SIZE 0 in my gfxconf.h, but I'm not sure if the work area size is actually used since I have not set GFX_USE_GTIMER to TRUE.
-
OK I'll keep you posted about my RA8875 touch panel driver. It is giving me good results already, it just needs to get a ugfx finish.
-
Is there some sort of walkthrough for writing a touch panel driver? I've found the examples in /boards/addons/ginput/touch and /drivers/ginput/touch, but they don't really align with the wiki page - there seems to be more to it than simply writing a file with an implementation of getpin_pressed(). All examples implement a board initialization function and some sort of read_xyz(), but I have not found any guide that tells me what to set in the passed GMouseReading struct and what to return (TRUE or FALSE), and why. What is the init sequence for the different modules? Is the ginput driver initialized before the gdisp driver? Where can I find that out?
-
Sometimes ugfx needs to measure the time difference between two events. These functions are wrappers around the HAL of your board, so something like this should do the trick: systemticks_t gfxSystemTicks(void) { return HAL_GetTick(); } systemticks_t gfxMillisecondsToTicks(delaytime_t ms) { return ms; // because 1 HAL tick is 1 ms as per the HAL reference manual } Edit: Oh I see you already have those. Well the purpose is simply to give ugfx a time base.
-
I'm trying to compile (without makefiles) the label example code found in the wiki (http://wiki.ugfx.org/index.php?title=Label). It seems that I need to add GEVENT, GQUEUE and GTIMER in addition to GINPUT, because the linker complains about undefined references to functions form those modules. In another thread it has been pointed out that GTIMER uses a separate thread, and now I'm really stuck. When I add those files from the ugfx source tree my linker stops complaining, which is fine. However, it has been noted in another thread that GTIMER uses a separate thread (viewtopic.php?f=22&t=191&p=1518&hilit=gtimer#p1518) which is simply not available in my environment. What can I do here?
-
Regarding the ugfx port to arduino - are you planning to do it like adafruit? They have separate libraries for the high-level API and each controller, which seems to work well. Others can simply write a controller library (like they can write one for ugfx now) and it will collaborate with a drawing interface.
-
One of the comments at aliexpress states that it works with u8glib and the ssd1106 driver - maybe have a look there?
-
So did you try the TinyScreen?